qpacketmodem
API Keywords: qpacketmodem modem modulate demodulate error correction encode decode
The qpacketmodem object conveniently combines linear modulation with interleaving , two levels of forward error-correction coding, and a data integrity check . The object combines the packetizer object with symbol packing for modulation. Both hard-decision and soft-decision decoding are supported, assuming the forward error-correction codec supports it.
Example Interface∞
Listed below is a simple example demonstrating the basic interface to the qpacketmodem encoder/decoder object:
#include <liquid/liquid.h>
int main() {
// options
modulation_scheme ms = LIQUID_MODEM_QPSK;
crc_scheme check = LIQUID_CRC_32;
fec_scheme fec0 = LIQUID_FEC_GOLAY2412; // inner
fec_scheme fec1 = LIQUID_FEC_NONE; // outer
unsigned int payload_len = 400; // number of bytes in message
// create and configure packet encoder/decoder objects
qpacketmodem enc = qpacketmodem_create(); // encoder
qpacketmodem dec = qpacketmodem_create(); // decoder
// configure
qpacketmodem_configure(enc, payload_len, check, fec0, fec1, ms);
qpacketmodem_configure(dec, payload_len, check, fec0, fec1, ms);
// get frame length
unsigned int frame_len = qpacketmodem_get_frame_len(enc);
// initialize payload
unsigned char payload_org[payload_len]; // original message
float complex frame_enc [ frame_len]; // encoded symbols
float complex frame_rec [ frame_len]; // received symbols
unsigned char payload_dec[payload_len]; // decoded message
// repeat as necessary
{
// ... initialize message ...
// encode message
qpacketmodem_encode(enc, payload_org, frame_enc);
// ... push through channel ...
// decode message and perform integrity check
int crc_pass = qpacketmodem_decode(dec, frame_rec, payload_dec);
}
// clean up objects
qpacketmodem_destroy(enc);
qpacketmodem_destroy(dec);
return 0;
}
Performance in AWGN∞
A useful metric for assessing the performance of a particular waveform is to analyze its ability to decode a packet in variouis channel environments. The figure below shows the performance of the qpacketmodem encoder/decoder in additive white Gauss noise channels:
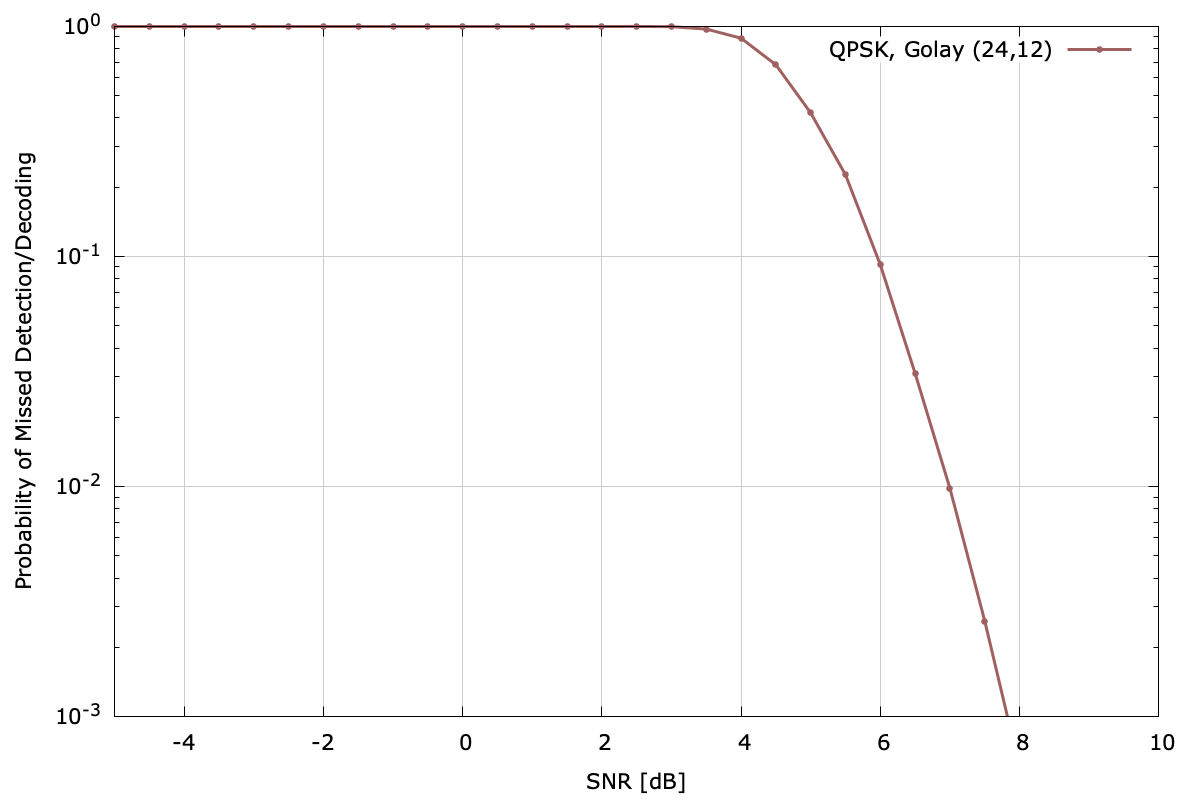
Performance of the qpacketmodem encoder/decoder in the presence of additive white Gauss noise with QPSK modulation and the Golay (24,12) codec .